January 29, 2023
My Docker Notes
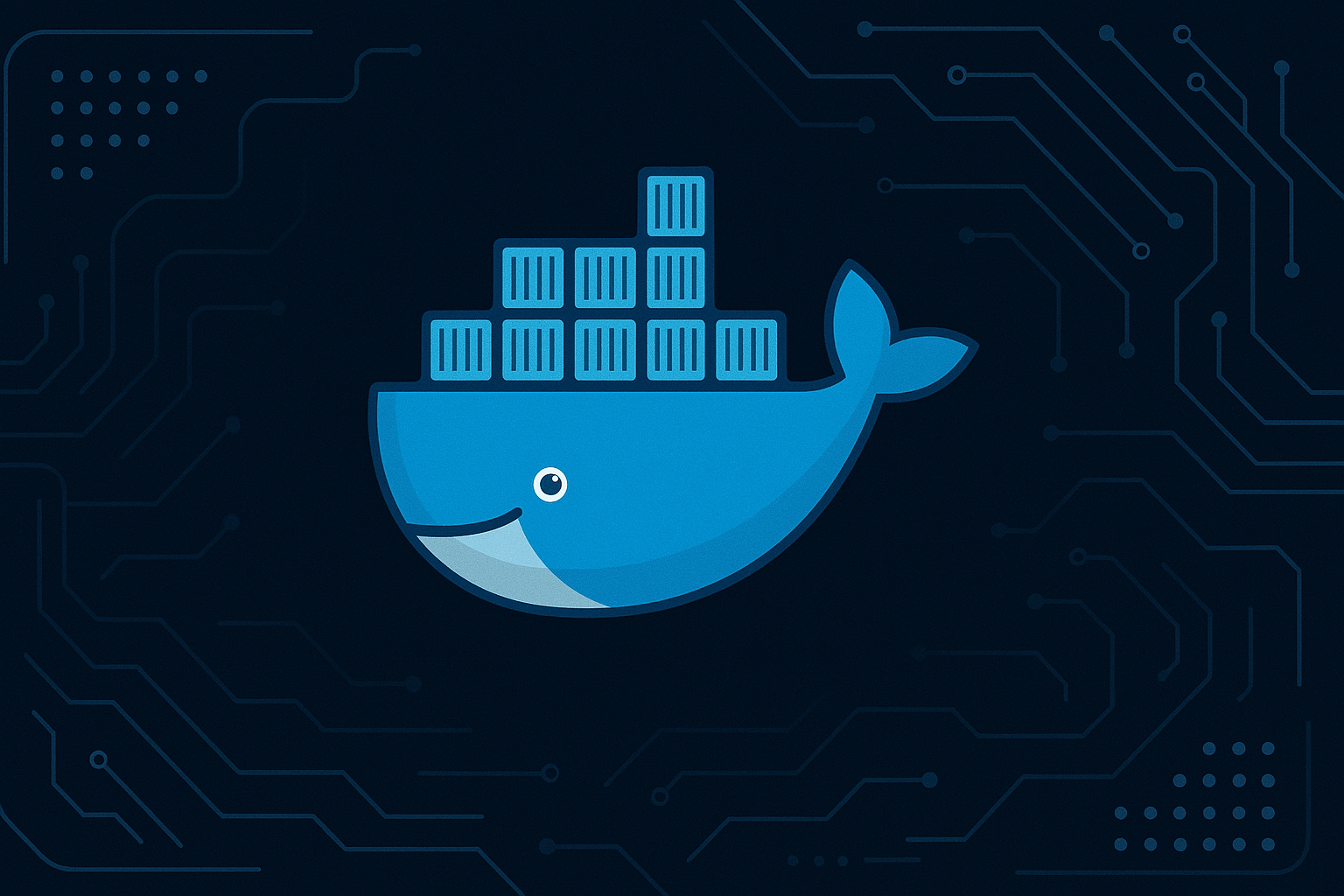
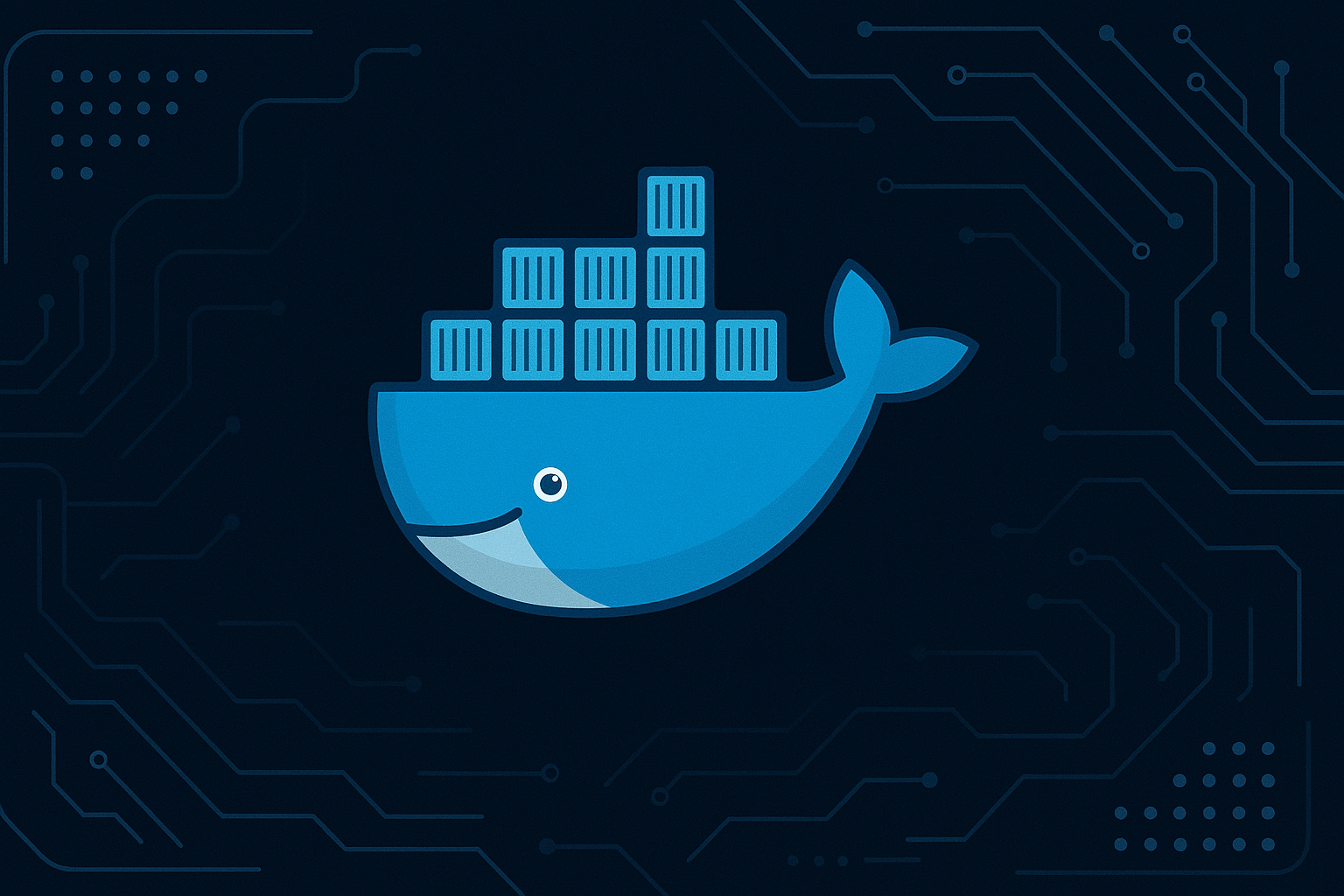
My Docker Notes
Docker is a powerful platform for developing, shipping, and running applications in containers. It ensures consistency across environments and simplifies deployment. Below is a detailed breakdown of essential Docker commands and concepts to help you manage containers efficiently.
Table of Contents
- Basic Docker Concepts and Theory
- Docker Networking
- Docker Volumes and Storage Architecture
- Image Management and Layer Concept
- Advanced Docker Concepts
- Docker Commands Cheat Sheet
- Conclusion
1. Basic Docker Concepts and Theory
Understanding Containers vs Images
- Images: Read-only templates containing application code, runtime, libraries and environment variables. Built from Dockerfiles.
- Containers: Runnable instances of images with a writable layer on top. Isolated processes with their own filesystem, networking, and process space.
Run a Container
The docker run
command combines several operations:
- Pulls the image if not locally available (
docker pull
) - Creates a new container (
docker create
) - Starts the container (
docker start
)
docker run <image_name>
Interactive Mode (-it flags)
-i
: Keeps STDIN open for interactive processes-t
: Allocates a pseudo-TTY (terminal) Together they create an interactive shell session. Essential for debugging or when containers need terminal input.
Container Lifecycle Management
- Detached mode (
-d
): Runs container in background - Stop vs Kill:
stop
: SIGTERM (graceful shutdown)kill
: SIGKILL (immediate termination)
- Persistent data: Stopped containers retain their filesystem changes until explicitly removed.
2. Docker Networking
Network Types Explained
-
Bridge Networks:
- Default network driver
- Containers get IPs in private subnet (172.17.0.0/16)
- NAT through docker0 interface
-
Host Networks:
- Bypasses network isolation
- Container uses host's network directly
- Better performance but less secure
-
Overlay Networks:
- Multi-host networking for Swarm
- Uses VXLAN encapsulation
DNS in Docker
- Containers can resolve each other by name within custom networks
- Embedded DNS server at 127.0.0.11
--link
is deprecated in favor of DNS-based discovery
Docker Networking Model
- Each container gets its own network namespace
- Default network drivers:
bridge
: Default network (NAT)host
: Shares host's network stacknone
: No networking
Port Publishing Mechanics
-P
: Auto-maps allEXPOSE
ports to random ephemeral ports (32768-60999)-p
: Explicit mapping (host_port:container_port)- Underlying technology:
- Linux: iptables rules
- Windows: NAT rules
3. Docker Volumes and Storage Architecture
Storage Drivers
Docker uses storage drivers to manage image layers and container writable layers:
overlay2
(default on Linux)aufs
devicemapper
zfs
Volume Types
-
Bind Mounts:
- Direct mapping to host filesystem
- Path must exist on host
- Performance varies by OS
-
Named Volumes:
- Managed by Docker
- Stored in
/var/lib/docker/volumes/
- Best for persistent data
-
tmpfs Mounts:
- In-memory storage
- Ephemeral (lost on container stop)
Data Flow
- Copy-on-Write (CoW) strategy for efficiency
- Union filesystem merges multiple layers
- Volumes bypass storage driver for direct I/O
4. Image Management and Layer Concept
Image Layers
- Each instruction in Dockerfile creates a layer
- Layers are cached for faster rebuilds
- Shared across images to save space
Build Process
- Parses Dockerfile
- Creates intermediate containers for each instruction
- Commits each step as new layer
- Tags final image
Registry Operations
- Push/pull uses content-addressable storage
- Image manifests reference layer digests
- Supports multi-arch images via manifest lists
5. Advanced Docker Concepts
Multi-Stage Builds
Docker's multi-stage build capability allows creating optimized production images by separating build-time dependencies from runtime requirements. The build process occurs in multiple phases where only the necessary artifacts are copied to the final image. This approach significantly reduces image size since build tools and intermediate files don't get included in the production image. For example, a Node.js application might use one stage with the full Node.js SDK for building, and then only copy the built JavaScript files to a lightweight nginx stage for serving.
# Build stage with full toolchain
FROM node:16 as builder
WORKDIR /app
COPY . .
RUN npm install && npm run build
# Production stage with only runtime
FROM nginx:alpine
COPY --from=builder /app/build /usr/share/nginx/html
Container Security Principles
Secure container deployment follows several key principles. First, containers should always run as non-root users whenever possible to limit potential damage from container breakout scenarios. Second, regular vulnerability scanning of images helps identify known security issues in dependencies. Third, careful management of build contexts through .dockerignore
files prevents accidental inclusion of sensitive files. These practices combine to create defense-in-depth for containerized applications.
FROM node:16
RUN useradd -m appuser && chown -R appuser /app
USER appuser # Principle of least privilege
BuildKit Architecture
Docker's BuildKit represents an evolution of the image building process, introducing parallel stage execution, improved cache efficiency, and advanced features like secret management. When enabled, it transforms the build process by creating a low-level build graph that can execute operations concurrently. The secret management feature allows securely passing credentials during build without leaving traces in the final image or intermediate layers.
# syntax=docker/dockerfile:1.3
FROM alpine
RUN --mount=type=secret,id=mysecret \
cat /run/secrets/mysecret > /config.txt
Container Health Monitoring
The healthcheck system provides a way for containers to self-report their operational status. This mechanism is particularly valuable in orchestrated environments where systems need to automatically detect and recover from failed containers. Health checks typically verify that application endpoints are responding or that internal processes are functioning correctly.
HEALTHCHECK --interval=30s --timeout=3s \
CMD curl -f http://localhost/health || exit 1
Resource Governance
Effective container deployment requires proper resource constraints to prevent any single container from monopolizing system resources. Docker allows setting memory limits, CPU shares, and other constraints that ensure fair resource allocation. These controls are especially important in multi-tenant environments where multiple containers share the same host.
docker run -it --memory="512m" --cpus="1.5" app-server
Orchestration Fundamentals
Docker provides native clustering through Swarm mode, which offers service discovery, load balancing, and rolling updates. The architecture consists of manager nodes that control the cluster and worker nodes that run containers. Services can be scaled horizontally, and the swarm handles distributing instances across available nodes.
docker swarm init
docker service create --name web --replicas 3 -p 80:80 nginx
Content Trust
Docker Content Trust implements digital signing of images using Notary, providing cryptographic verification of image authenticity. When enabled, it ensures that only signed images can be pulled or run, protecting against tampering in the supply chain. This is particularly valuable for production environments where image integrity is critical.
export DOCKER_CONTENT_TRUST=1
docker pull organization/trusted-image
6. Docker Commands Cheat Sheet
Container Management
Command | Description |
---|---|
docker run <image> | Run a container from an image |
docker run -it <image> /bin/bash | Run container interactively with shell access |
docker run -d <image> | Run container in detached mode |
docker run --name <name> <image> | Run container with custom name |
docker ps | List running containers |
docker ps -a | List all containers (including stopped) |
docker start <container> | Start a stopped container |
docker stop <container> | Stop a running container gracefully |
docker restart <container> | Restart a container |
docker kill <container> | Force stop a container |
docker rm <container> | Remove a stopped container |
docker rm -f <container> | Force remove a running container |
docker container prune | Remove all stopped containers |
docker exec <container> ls | Run a command inside a running container |
Image Management
Command | Description |
---|---|
docker images | List all images |
docker pull <image> | Download an image from registry |
docker push <image> | Push an image to registry |
docker rmi <image> | Remove an image |
docker image prune | Remove unused images |
docker build -t <name> . | Build image from Dockerfile |
docker history <image> | Show image layers |
docker tag <image> <new_tag> | Tag an image |
Networking
Command | Description |
---|---|
docker network ls | List networks |
docker network create <name> | Create a network |
docker network inspect <name> | Inspect a network |
docker network connect <network> <container> | Connect container to network |
docker network disconnect <network> <container> | Disconnect container from network |
docker network prune | Remove unused networks |
Volumes & Storage
Command | Description |
---|---|
docker volume ls | List volumes |
docker volume create <name> | Create a volume |
docker volume inspect <name> | Inspect a volume |
docker volume rm <name> | Remove a volume |
docker volume prune | Remove unused volumes |
docker run -v /host/path:/container/path <image> | Bind mount host directory |
docker run -v volume_name:/container/path <image> | Mount named volume |
Port Mapping
Command | Description |
---|---|
docker run -p 8080:80 <image> | Map host port 8080 to container port 80 |
docker run -P <image> | Auto-map all exposed ports to random ports |
docker port <container> | Show port mappings for container |
Logs & Monitoring
Command | Description |
---|---|
docker logs <container> | View container logs |
docker logs -f <container> | Follow logs in real-time |
docker stats | Show live container resource usage |
docker top <container> | Show running processes in container |
docker events | Show real-time events from server |
System & Cleanup
Command | Description |
---|---|
docker info | Display system-wide information |
docker version | Show Docker version |
docker system df | Show disk usage |
docker system prune | Remove unused data (images, containers, networks) |
docker system prune -a | Remove all unused data including dangling images |
Docker Compose
Command | Description |
---|---|
docker-compose up | Create and start containers |
docker-compose up -d | Start containers in detached mode |
docker-compose down | Stop and remove containers |
docker-compose ps | List containers |
docker-compose logs | View output from containers |
docker-compose build | Build images |
docker-compose exec <service> <command> | Execute command in running service |
7. Conclusion
Docker simplifies application deployment by encapsulating environments in containers. Mastering these commands will help you efficiently manage containers, networks, and volumes.
By understanding these Docker fundamentals, you can streamline your development and deployment workflows. Happy Dockering! 🐳
Thank you for reading! I hope you found this post insightful. Stay curious and keep learning!
📫 Connect with me:
© 2025 Ayush Rudani